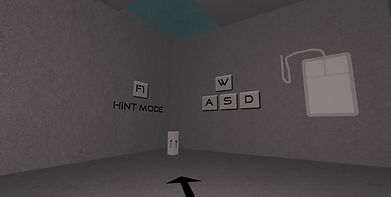
Warpalorpadingdong is a silly-named first person puzzle-platformer I created in Unity as part of my senior thesis at Mt Sierra College. Aside from the first-person character controller script from Unity's built-in libraries, all scripts and assets were done by me. On this page you can read about the design of the game as well as see samples of the scripting that went into making it.
You will need to unzip the folder before running the application.
Design
Warpalorpadingdong's design originates from the idea of first person platforming by shooting. Basically players utitlize 4 different guns that essentially teleport them where they shoot, and 1 that pulls them like a tractor beam. Players only hold 1 gun at a time and gun pickups are placed to serve the puzzles. There are also gravity manipulation switches throughout the game's level to spice up the puzzles by making walls and ceilings into floors and floors into walls and ceilings.
The platforming-by-shooting came to me after seeing reviews of Airtight Games' Quantum Conundrum criticize the game's first person platforming for being clumsy. I theorized that an advantage of Valve's Portal, the game that popularized physics-based first person puzzle games, was that it still played to the first person perspective's strenghts by heavily involving shooting a gun, just with a completely different context from attacking enemies. I wanted to try taking this line of thinking to what I feel is the logical extreme by making a game where shooting wasn't just an aid in traversal, it is a direct means of traversal.
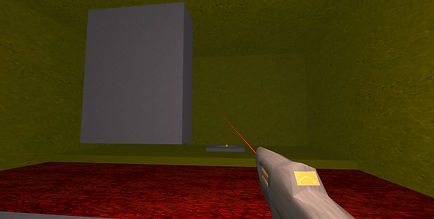
With that focus on shooting, I decided pretty much immediately that I'd need different guns to help keep the puzzles and challenges fresh. I bounced a lot of ideas around in my head, but I wanted to make sure that I didn't end up with any that felt too similar to one another. The 5 I ended up fleshing out and implementing are as follows. The arcshot, as it's name implies, fires its projectile in a grenade-like arc, and when it impacts a surface, the player teleports to where it hit. Keeping with the clever theme-naming, the instashot teleports the player where it's pointed as soon without waiting for a projectile to travel. Not only is it easy to use, it can also bypass deadly transparant red volumes. The slowshot is the most situational of the guns, but in a puzzle game players still need to be ready to use any and all of their tools. It fires a slow moving projectile in a straight line that teleports the player to it on impact. The benefit is that it lets players flip switches, swap guns, or execute whatever other setup they may need before the teleport happens. If I recall correctly, the bounceshot was the first gun variation I thought of. It fires a projectile that fires in a straight line and teleports the player to it on the second impact, after bouncing off on the first. Finally the magshot is the most unique weapon of the 5. It instantly creates a magnet wherever it's pointed that pulls the player straight towards it until they reach it or cancel the shot by pulling the trigger again.
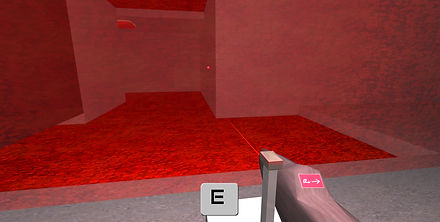
The heavy use of gravity manipulation came about because I felt it would create interesting level design to have players revisiting the same areas with flipped gravity that completely changes the experience from when they first entered. I'm not alone in finding that kind of interconnectivity in level design fascinating in other games. Before I designed the full level for the game I experimented with the gravity mechanic in my test scene and determined that not only would it be doable within the scope of the project, it seemed to mesh well with the other mechanics. In hindsight it may have ended up overshadowing the teleporting mechanices that were meant to be the core of the game in some ways, but that's sometimes how game development goes.
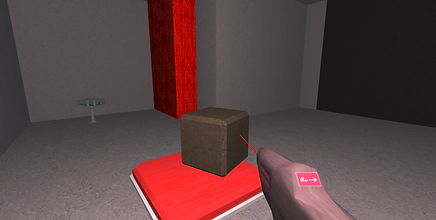
Partly due to the technical problems that came about from gravity flipping and box carrying and whatnot, and partly due the other aspects of my senior thesis that needed my time, Warpalorpadingdong is a lot more minimalistic than my other main thesis project, Elemental Bots. However, while things like visuals and presentation may be be lacking compared to Elemental Bots, I was able to polish the core gameplay to reliably function as it should, which was my primary focus when finishing it up. Finally, in case you're wondering about the name: I was talking about that with a couple of my friends at school and said something like, "Well, I could name it something really stupid like 'Warpalorpadingdong'" (because warping around is the original design focus). Naturally they pressured me into actually calling it that and I don't really regret it because it is pretty funny to naturally say in conversation.
Scripting
Warpalorpadingdong was coded entirely in Unity's version of Javascript. You can view a sampling of the scripts used in the zip file linked above. The scripts can be viewed in any program that can read .js files, such as Notepad++. Some further notes and explanation for each of them follows.
Ballshot
This script is attatched to the objects fired by the 3 projectile-based guns (arc shot, slow shot, and bounce shot). The "OnCollisionEnter" and "OnTriggerEnter" functions are automatically called when the ball reaches a surface or volume. If the ball reaches an acceptable surface for warping with no remaining bounces, the "hasHit" boolean is set so the update loop will execute the teleportation code after the appropriate delay. When the hit is ready, the "Update" function checks the clearance of the shot in all 6 directions and pushes the ball to a space with enough clearance for the player to teleport without clipping through walls. If no such space exists the shot is canceled, otherwise the player is moved to where the ball is.
Grab
Picking up boxes to hold down buttons is a mechanic in the middle section of the level. This script handles letting the player pick up and drop said boxes. The script uses a raycast to see if the player is looking at a box they are close enough to grab, then makes that box a child of the player (more specifically the camera) if the player presses the "use" key. The object is then dropped if the use key is pressed again of the box is pushed out of reach. Originally I tried to make this script more complicated by having the object contstantly trying to push itself to the center of view, but after difficulty getting the physics and collisons bug-free I tested it with this simpler setup and found the gameplay still worked just as well even if the box could easily be pushed off center.
Gun
This script handles all the players guns, which are actually just one gun that changes material and behavior. When the player presses the fire button the gun creates the appropriate shot object, or cancels the current shot if there is one. The insta shot and mag shot check the surface they'll hit with a raycast and then instantiate at the point of impact (where the the insta shot uses similar checks to the ball shot's to get into a clear space before teleporting), while the ball shots are instantiated at the gun and given the appropriate force based on the shot type index. The "raised" boolean was meant to raise the gun to face straight up to avoid it clipping into a wall if the player got too close to it, and while the visuals for that didn't make it into the game due to time constraints, the gun still becomes disabled if it's clipping into the wall so the player can't fire out of bounds.
GunPickUp
The pedestals where the player picks up and switchs gun have this script. Aside from switching the index of the player's current gun when the player interacts with the pedestal, the pedestal also enables or disables the gun model sitting on it based on whether or not the player already has that gun type.
Rotate
This script is for the switches that flip gravity. Because I'm using Unity's character controller object and physics engine that are set up with the assumption that negative on the y-axis is always down, I found early on that the best approach was not to rotate the player, but to rotate everything but the player. To rotate everything with the current switch as a pivot, I temporarily make virtually everything in the scene a child of the switch, then rotate the switch before reverting everything to not being children of the switch. One of the first problems I encountered was that the rotation would fling the player off in some direction away from the switch, so to solve that I made the switch prefab surrounded on all sides by invisible walls that are only solid for a half second after the switch is activated. The player still gets sent flying, but they are stopped while they're still by the switch so it ends up not being noticible with the gravity flipping as a distraction. The other problem was maintaining the correct velocity of shots that were in flight when a gravity flip happens (which is used for a puzzle). I found that the most reliable solution was to zero the shot's velocity at the start of a flip, then give it a new velocity of the same magnitude in the new direction it's facing after it has been rotated (this works since shots don't rotate under other circumstances).